1.9. ChatGPT#
You may well have heard of ChatGPT, an AI tool that is uncannily good at writing English.
What you may not know, is that whilst ChatGPT is good at writing English, it is really good at writing code. Basically it reproduces things people have posted on the internet, and there is a lot of code on the internet, especially in Python.
You can use ChatGPT to help you write code, as you will see in the example below.
The existance of ChatGPT does not mean it is pointless to learn Python! Quite the opposite!
Chat GPT means that if you learn a little coding - enough coding concepts and syntax to read code, you will be able to write code easily with help of ChatGPT.
In other words:
In days gone by, if you took a basic coding course (like this one) you could write basic code
Now, with help of AI, if you take a basic coding course like this one you can write advanced and efficient code
1.9.1. Is it cheating?#
No.
Coders have always worked by taking existing code (traditionally, written by other humans) and modifying it. This is how you learn to code, and how most code for practical applications is developed. For example, many research labs share all their code via an online repository called GitHub; new lab members will develop their data analysis code based on what other lab members have done in the past.
In this course, I encourage you to use the code snippets in my worked examples to help you complete the exercises and assignments. You need to understand them so you can modify them as needed, but you don’t need to write them from scratch.
Using code generated (or basically, found on the internet) by ChatGPT is no different from the above examples really and is an acceptable way to procede, but you should (in all cases where you are modifying existing code), try to understand what the code, otherwise you will end up with your code not doing what was intended.
One caveat to be aware of is that at present it is forbidden to use ChatGPT in University exams, which would include the exam for this course. It is however permitted to use your own notes and the course notes (i.e. this website) in the exam for this course, so you will not be examined on writing code from scratch, but rather sourcing and modifying suitable code.
1.9.2. Example#
I decided to see what ChatGPT would make of the coin-toss example.
If you have a ChatGPT account (you can make one for free) you can try this for yourself.
We are going to want to copy the code into the Jupyter Notebook and run it, so let’s set up our Python libraries as usual:
# Set-up Python libraries - you need to run this but you don't need to change it
import numpy as np
import matplotlib.pyplot as plt
import scipy.stats as stats
import pandas as pd
import seaborn as sns
sns.set_theme(style='white')
import statsmodels.api as sm
import statsmodels.formula.api as smf
Ask your question in English#
I started by asking ChatGPT for some code:
Note that ChatGPT not only writes the code, but it kindly explains what it has done in English afterwards!
Run it!#
Let’s copy-paste the code into our notebook and run it. Since the code you see above is actually a screenshot, I have pasted the text for you - but if you work with ChaatGPT directly you can copy-paste its output into a Jupyter notebook just like any other text.
Try running the code below a few times and watch how the output changes each time
Think - how is the output from this code different from the code my example, in section 1.6.1 of these notes?
import random
# Initialize counters for heads and tails
heads_count = 0
tails_count = 0
# Number of times to toss the coin
num_tosses = 10
# Simulate tossing the coin 10 times
for _ in range(num_tosses):
# Generate a random number (0 or 1) to represent heads or tails
coin_toss = random.randint(0, 1)
# Check the result and update the respective counter
if coin_toss == 0:
heads_count += 1
else:
tails_count += 1
# Display the results
print(f"Heads: {heads_count}")
print(f"Tails: {tails_count}")
Heads: 6
Tails: 4
Understanding the code#
I’m now going to try running little bits of the code separately to get a better understanding of it.
Import necessary libraries#
First, I’m going to import the same packages that Chat GPT used - checking at the top of the code snippet to see what they were.
Note that in the example Jupyter notebooks in these course notes, there is always a block at the top importing the relevant Python libraries which are the ones used in my code on this course; but ChatGPT doesn’t limit itself ot the same set of libraries, so you might need to import any additional ones it is using.
import random
Try out any unfamiliar functions#
According to the explanatory note, ChatGPT used a function called random.randint()
, and indeed I can see that this was run on each pass through the loop.
I’m not familiar with the function random.randint()
so I might have a little try in my Jupyter notebook to see what it does (it could also be useful to Google for the documentation of this function, although in this case I think that won’t be necessary)
Since we know (from the name and the explanatory text) that it is a random function, we might try running it a few times to see if the output changes randomly.
random.randint(0,1)
0
OK, it generates 0s and 1s at random.
Comprehension questions#
The result of running ChatGPT’s code is a count of how many heads and tails we got (from 10 coin tosses)
This is different from my version in section 1.6.1 of these note, which also saved each individual outcome in an array, and hence retains information about the order of the outcomes
What appraoch did ChatGPT use to count the heaads, which resulted in it retaining only the total number of heads but not the order of heads and tails?
This may be unfamiliar syntax but look at the code and have a guess
1.9.3. Modifying the question#
ChatGPT did exactly what I asked (counted the number of heads) but I have not realised I actually wanted to record the sequence of heads and tails. How can I modify my question to get nearer to what I want?
Here’s my next attempt:
I started by asking ChatGPT for some code:
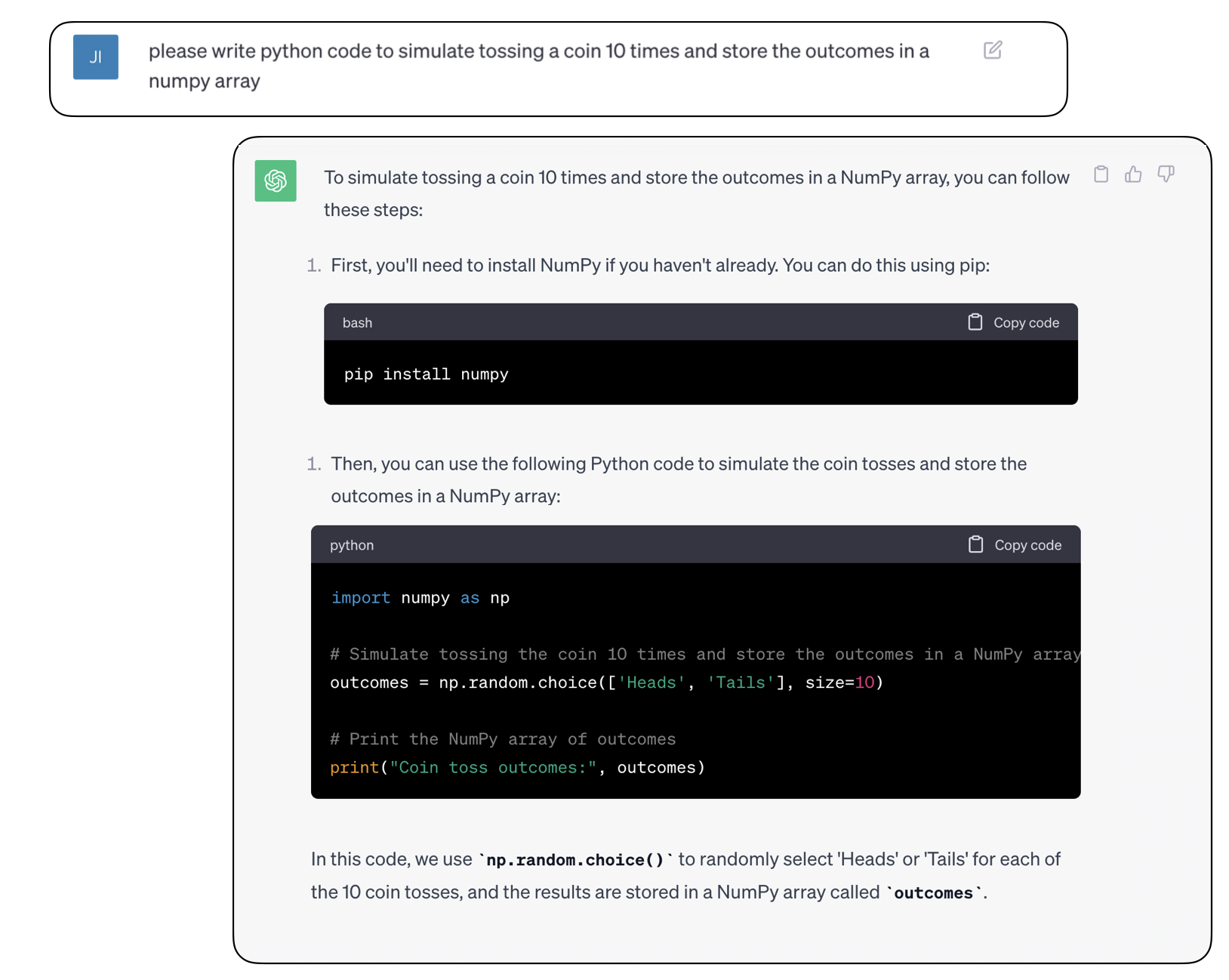
Ooh, it looks like this is a little more efficient than my version, as ChatGPT has realised there is a numpy
function to generate the array of 10 ‘coin tosses’ in one step, rather than going round a loop 10 times.
Let’s check it out on the command line
We won’t need to install or import numpy
as we already have this (it is imported int he code block aat the top fo this notebook) so we can get straight down to business:
outcomes = np.random.choice(['Heads','Tails'], size=10)
print("Coin toss outcomes:", outcomes)
Coin toss outcomes: ['Heads' 'Heads' 'Heads' 'Heads' 'Tails' 'Heads' 'Tails' 'Heads' 'Tails'
'Heads']
Uh-oh, I’m not going to be able to count the heads by summing the array, am I?
I’d rather we coded heads and tails as 0 and 1.
I could go back and ask ChatGPT again, but maybe I could just slightly change the code to do that?
outcomes = np.random.choice([0,1], size=10)
print("Coin toss outcomes:", outcomes)
Coin toss outcomes: [0 1 1 0 0 1 1 0 0 1]
sum(outcomes)
5
Hurrah!
Comprehension question#
What is wrong with the following code?
What would happen if I try to count the heads by taking the sum of the outcomes?
outcomes = np.random.choice(['0','1'], size=10)
print("Coin toss outcomes:", outcomes)
Coin toss outcomes: ['1' '1' '0' '1' '1' '1' '1' '0' '1' '0']
1.9.4. Conclusion#
Chat GPT can really help with this coding task, but you may need to make some tweaks to get exactly what you want.
It can be very helpful, BUT in order to make use of it you will need to be able to read the code it outputs - this course should give you that ability.
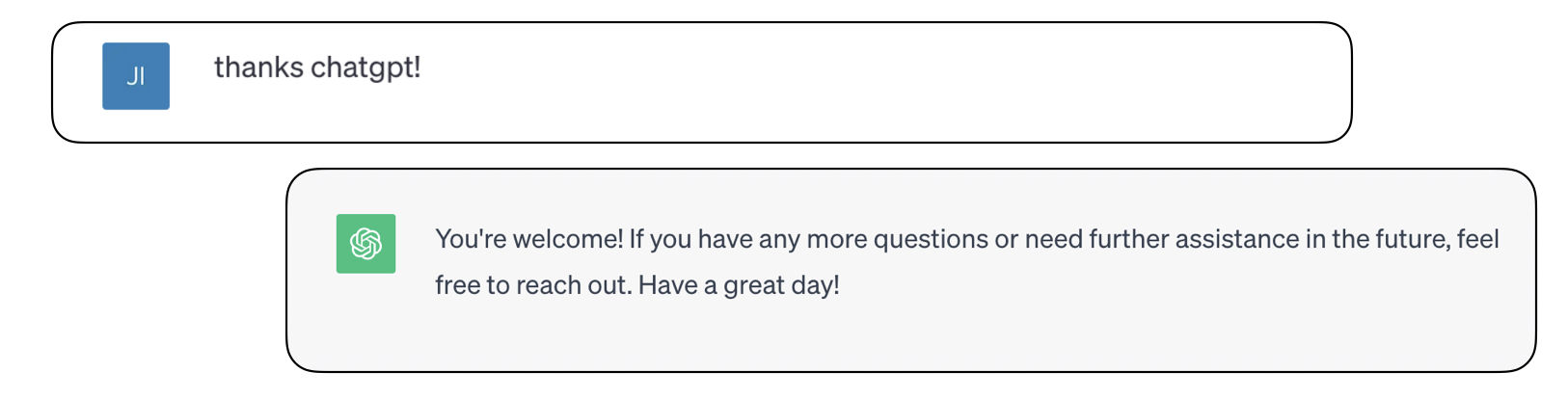
Aw, what a ray of sunshine.
I don’t think it’s plotting to take over the world and enslave us all, do you?
Thanks to David Hays for suggesting this exercise