1.5. The FOR loop#
This page (and subsequent pages) is a Jupyter Notebook. Download it or open it in Colab so you can actually tun the code blocks!
The thing computers are really good at is repeating a calculation lots of times
One way to do this is using a loop
Here we meet the for
loop (there is also such thing as a while
loop which we will meet later)
1.5.1. Set up Python libraries#
As usual, run the code cell below to import the relevant Python libraries
# Set-up Python libraries - you need to run this but you don't need to change it
import numpy as np
import matplotlib.pyplot as plt
import scipy.stats as stats
import pandas as pd
import seaborn as sns
sns.set_theme(style='white')
import statsmodels.api as sm
import statsmodels.formula.api as smf
1.5.2. My first loop#
Say we want the computer to repeat a task a few times - in this casae, the task is to say “hello” three times.
We can get the computer to do this using a for loop.
The
for
loop is controlled by a counter variable i.i counts how many times we have been round the loop (in this case, how many times we have said “hello”).
When we have repeated the loop the required number of times, we stop
for i in [0,1,2]:
print(str(i) + ": hello!")
0: hello!
1: hello!
2: hello!
Comprehension questions#
a. See if you can change the code to say “hello” five times
1.5.3. Setting the number of iterations#
The number of iterations is the number of times we want to go round the loop
Imagine if we wanted to run the loop 100 times, it would be a bit annoying to write out all the values of i we want to use
for i in [0,1,2,3,4,5,6 ......... 100]
luckily we can generate this list automatically using range()
for example
for i in range(5)
has the same effect as
for i in [0,1,2,3,4]
let’s try it!
for i in range(5):
print(str(i) + ": hello!")
0: hello!
1: hello!
2: hello!
3: hello!
4: hello!
1.5.4. Changing the input on each iteration#
Our counter variable i can also be used to count through a list of inputs.
Say we want to say hello to three of our friends:
friends = ['Alan','Barry','Craig']
for i in range(3):
print(str(i) + ": Hello " + friends[i] + "!")
0: Hello Alan!
1: Hello Barry!
2: Hello Craig!
Look at the print statement. friends[i]
is getting the name from position i in our input list, friends
Comprehension Question#
a. What would happen if I run the code block below?
Think first, and then uncomment and run the code to check!
# print(friends[2])
b. The code block below will give an error. Why?
# print(friends[4])
Automatically set number of iterations#
To avoid errors, we can autoamtically set the maximum value of i to match the length of our input list, using the function len()
len(friends)
3
Putting it together, we can do:
friends = ['Alan','Barry','Craig']
for i in range(len(friends)):
print(str(i) + ": Hello " + friends[i] + "!")
0: Hello Alan!
1: Hello Barry!
2: Hello Craig!
Comprehension questions#
Say I add some friends to my list:
friends = ['Alan','Barry','Craig','David','Egbert']
a. What will len(friends)
be now?
b. What will happen if I run the code block below?
This about what you expect to happen first
Then uncomment and run the code block to test your hypothesis
#friends = ['Alan','Barry','Craig','David','Egbert']
#for i in range(3):
# print(str(i) + ": Hello " + friends[i] + "!")
c. Can you fix the code block in part b?
d. What will happen if I run the code block below?
This about what you expect to happen first
Then uncomment and run the code block to test your hypothesis
#friends = ['Alan','Barry','Craig']
#for i in range(5):
# print(str(i) + ": Hello " + friends[i] + "!")
e. Why do you think that happened? Can you fix the code block in part d?
1.5.5. Saving the output on each iteration#
Sometimes we don’t just want to print an output to the screen!
Instead we would like to save the outputs in a list or array so we can use them later (eg, plot them on a graph)
If we want to do this we need to create an empty list or array to store them in. For our purposes, we will many be working with numbers, so a numpy
array is a good choice
Example
Let’s make a little loop that takes the numbers 0-5 and squares them:
x = [0,1,2,3,4,5]
for i in range(len(x)):
print(str(x[i]) + ' squared is ' + str(x[i]*x[i]))
0 squared is 0
1 squared is 1
2 squared is 4
3 squared is 9
4 squared is 16
5 squared is 25
Here we have an array of inputs - the values of x. On each loop we call the value from position i in this array using the syntax: x[i]
Note the similarity to the syntax
friends[i]
in the loop above
Comprehension questions#
a. What is x[4]
Think then check!
Don’t forget in Python we count from zero!
# print(str(x[4]))
Now instead of printing the numbers to the screen, we create an array to save them in using np.empty()
:
squares = np.empty(len(x))
This created an empty numpy
array called squares with the same number of ‘slots’ as our input array, x
We can then fill these ‘slots’ in squares one by one on each pass through the loop
x = [0,1,2,3,4,5]
squares = np.empty(len(x))
for i in range(len(x)):
squares[i] = x[i]*x[i]
print(squares)
[ 0. 1. 4. 9. 16. 25.]
Now we have saved our output, instead of just putting it on the screen, we can do other things with it, for example plot it:
don’t worry about the syntax for plotting, this is covered in a later session
plt.plot(x,squares, 'k.-')
plt.xlabel('$x$'), plt.ylabel('$x^2$')
plt.show()
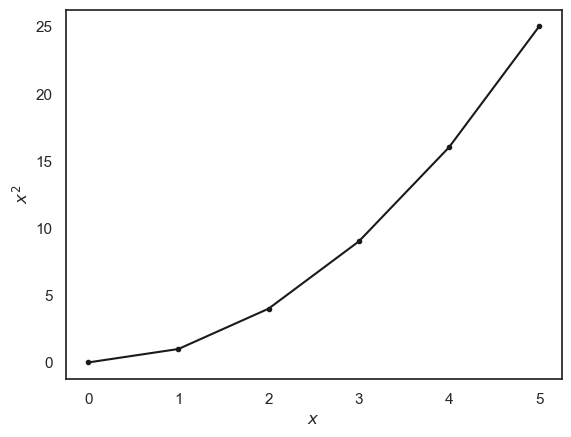
Comprehension questions#
a. When i==2
, what are x[i]
and squares[i]
?
Think then check!
Don’t forget we count from zero in Python (goodness knows why!)
#i=2
#print(x[i])
#print(squares[i])
1.5.6. Exercise: Fibonacci Sequence#
Let’s try out our newly learned skills by making a loop to generate the Fibonacci sequence
The Fibonacci Sequence is a sequence of numbers that begins [0,1,…] and continues by adding together the two previous numbers such that
The sequence begins [0, 1, 1, 2, 3, 5, 8, 13….. ] and goes on forever.
You can find some fun facts about the Fibonacci sequence here:
Let’s make a for
loop to calculate the first 10 elements of the Fibonacci sequence.
Uncomment and complete the code below
#n = 10 # number of elements to calculate
#fibonaccis = np.empty(n)
## get the sequence started
#fibonaccis[0] = 0
#fibonaccis[1] = 1
#for i in range(2,n): # start in place 2 as we already set the first two elements of the sequence (places 0 and 1)
# fibonaccis[i] = # your code here to calculate the ith Fibonacci number
## This bit will plot the Fibonacci sequence
#plt.plot(fibonaccis,'.-')
#plt.xlabel('$i$')
#plt.ylabel('$x_i$')